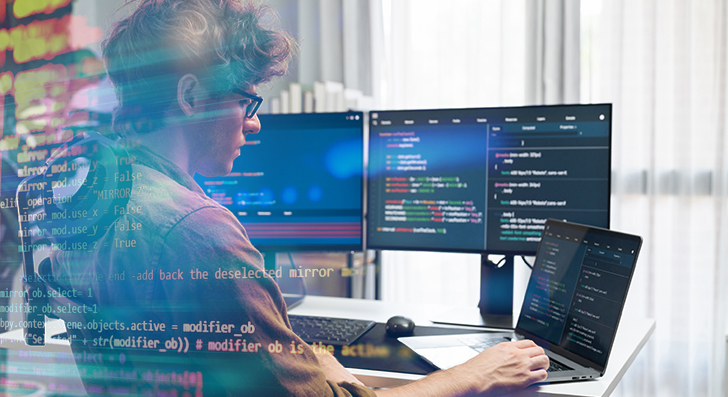
Scalability usually means your application can handle advancement—additional consumers, much more knowledge, and a lot more site visitors—without breaking. To be a developer, constructing with scalability in mind will save time and tension later. Right here’s a transparent and useful guide to assist you to start off by Gustavo Woltmann.
Design for Scalability from the beginning
Scalability isn't a thing you bolt on later on—it should be portion of your system from the beginning. Quite a few applications fall short when they increase quickly because the initial structure can’t manage the additional load. As being a developer, you'll want to Believe early about how your method will behave stressed.
Start by developing your architecture to generally be flexible. Keep away from monolithic codebases where by every little thing is tightly related. As an alternative, use modular design or microservices. These designs split your application into smaller sized, impartial sections. Each module or support can scale By itself with out impacting The full procedure.
Also, consider your database from day one particular. Will it have to have to handle a million people or simply just a hundred? Choose the correct sort—relational or NoSQL—determined by how your details will grow. Plan for sharding, indexing, and backups early, even if you don’t will need them nonetheless.
Another essential level is in order to avoid hardcoding assumptions. Don’t publish code that only performs underneath present-day conditions. Take into consideration what would transpire If the person foundation doubled tomorrow. Would your app crash? Would the database slow down?
Use style patterns that support scaling, like information queues or occasion-driven systems. These help your application tackle additional requests devoid of finding overloaded.
If you Create with scalability in your mind, you're not just getting ready for success—you might be lessening long run complications. A effectively-planned procedure is easier to take care of, adapt, and improve. It’s greater to organize early than to rebuild later.
Use the ideal Databases
Selecting the right databases can be a critical Section of developing scalable applications. Not all databases are designed precisely the same, and using the Completely wrong one can slow you down or maybe result in failures as your app grows.
Start by being familiar with your info. Is it remarkably structured, like rows within a desk? If Indeed, a relational databases like PostgreSQL or MySQL is an efficient in shape. They are potent with associations, transactions, and regularity. Additionally they aid scaling tactics like read replicas, indexing, and partitioning to manage much more website traffic and knowledge.
In case your facts is more versatile—like person activity logs, product or service catalogs, or documents—look at a NoSQL alternative like MongoDB, Cassandra, or DynamoDB. NoSQL databases are improved at handling significant volumes of unstructured or semi-structured info and will scale horizontally more simply.
Also, consider your go through and produce patterns. Do you think you're accomplishing plenty of reads with much less writes? Use caching and read replicas. Have you been managing a heavy create load? Investigate databases which can deal with substantial produce throughput, or even celebration-centered data storage methods like Apache Kafka (for short term facts streams).
It’s also good to Believe ahead. You may not need to have State-of-the-art scaling options now, but selecting a database that supports them signifies you gained’t will need to modify afterwards.
Use indexing to hurry up queries. Stay clear of unnecessary joins. Normalize or denormalize your information according to your entry styles. And normally monitor databases performance when you grow.
In short, the right databases relies on your application’s composition, speed needs, And exactly how you hope it to mature. Choose time to select correctly—it’ll preserve a great deal of problems later.
Optimize Code and Queries
Quickly code is key to scalability. As your application grows, each individual smaller delay adds up. Poorly penned code or unoptimized queries can decelerate functionality and overload your program. That’s why it’s vital that you Develop effective logic from the beginning.
Start out by composing thoroughly clean, simple code. Stay clear of repeating logic and take away anything at all needless. Don’t choose the most advanced Resolution if an easy 1 is effective. Maintain your functions small, targeted, and easy to check. Use profiling tools to uncover bottlenecks—spots exactly where your code usually takes far too extended to operate or employs an excessive amount of memory.
Future, examine your databases queries. These usually gradual factors down more than the code by itself. Make sure each query only asks for the info you actually will need. Stay away from Find *, which fetches every little thing, and in its place pick precise fields. Use indexes to speed up lookups. And keep away from doing too many joins, especially across substantial tables.
In the event you observe the same info staying requested repeatedly, use caching. Retail store the outcomes briefly working with equipment like Redis or Memcached this means you don’t have to repeat pricey functions.
Also, batch your databases functions when you can. As opposed to updating a row one after the other, update them in teams. This cuts down on overhead and will make your app much more productive.
Make sure to test with big datasets. Code and queries that operate high-quality with a hundred documents might crash after they have to deal with 1 million.
In a nutshell, scalable applications are rapid applications. Keep the code tight, your queries lean, and use caching when needed. These actions aid your application remain easy and responsive, whilst the load will increase.
Leverage Load Balancing and Caching
As your app grows, it's to deal with a lot more consumers and even more targeted visitors. If all the things goes as a result of one server, it will quickly turn into a bottleneck. That’s where by load balancing and caching are available. Both of these equipment assist keep your application speedy, secure, and scalable.
Load balancing spreads incoming traffic throughout many servers. In place of one particular server undertaking each of the function, the load balancer routes customers to different servers dependant on availability. What this means is no solitary server gets overloaded. If one server goes down, the load balancer can send out visitors to the Other folks. Resources like Nginx, HAProxy, or cloud-based methods from AWS and Google Cloud make this very easy to create.
Caching is about storing data quickly so it may be reused quickly. When buyers request exactly the same information and facts once again—like a product web page or simply a profile—you don’t ought to fetch it in the databases each and every time. You can provide it in the cache.
There's two frequent types of caching:
1. Server-facet caching (like Redis or Memcached) merchants data in memory for rapid entry.
two. Consumer-facet caching (like browser caching or CDN caching) retailers static data files close to the person.
Caching minimizes databases load, improves pace, and makes your application more productive.
Use caching for things which don’t alter generally. And usually ensure that your cache is updated when facts does change.
In a nutshell, load balancing and caching are very simple but effective instruments. Together, they help your application handle a lot more people, stay quickly, and recover from difficulties. If you intend to mature, you will need both equally.
Use Cloud and Container Applications
To construct scalable programs, you require applications that let your app expand quickly. That’s where cloud platforms and containers come in. They give you adaptability, reduce setup time, and make scaling A lot smoother.
Cloud platforms like Amazon World wide web Expert services (AWS), Google Cloud System (GCP), and Microsoft Azure Permit you to hire servers and services as you'll need them. You don’t must get hardware or guess foreseeable future ability. When website traffic improves, you could include a lot more assets with only a few clicks or instantly employing automobile-scaling. When targeted traffic drops, it is possible to scale down to save cash.
These platforms also supply companies like managed databases, storage, load balancing, and protection equipment. It is possible to target creating your app rather than managing infrastructure.
Containers are Yet another important tool. A container offers your application and almost everything it has to run—code, libraries, configurations—into just one unit. This makes it quick to maneuver your app between environments, from a laptop computer for the cloud, with out surprises. Docker is the most popular tool for this.
Once your app uses many containers, equipment like Kubernetes assist you to manage them. Kubernetes handles deployment, scaling, and Restoration. If a single part of your respective app crashes, it restarts it quickly.
Containers also help it become simple to different areas of your application into companies. You are able to update or scale components independently, which happens to be great for general performance and dependability.
In short, employing cloud and container tools suggests you are able to scale rapid, deploy very easily, and Get better rapidly when challenges take place. If you prefer your app to increase with out limitations, start out employing these applications early. They preserve time, cut down danger, and make it easier to stay focused on making, not correcting.
Check Anything
If you don’t keep an eye on your software, you won’t know when issues go Mistaken. Checking helps you see how your app is undertaking, location troubles early, and make improved decisions as your app grows. It’s a crucial Section of setting up scalable systems.
Begin by tracking standard metrics like CPU utilization, memory, disk House, and reaction time. These show you how your servers and services are carrying out. Equipment like Prometheus, Grafana, Datadog, or New Relic can assist you accumulate and visualize this details.
Don’t just observe your servers—monitor your app also. Control just how long it requires for end users to load web pages, how frequently glitches transpire, and wherever they come about. Logging instruments like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly may help you see what’s occurring within your code.
Put in place alerts for critical troubles. By way of example, When your response time goes over a limit or a service goes down, you should get notified straight away. This assists you fix issues speedy, generally ahead of consumers even discover.
Monitoring is usually handy if you make adjustments. If you deploy a completely read more new element and see a spike in errors or slowdowns, you could roll it back again prior to it causes authentic hurt.
As your app grows, targeted visitors and knowledge improve. With out checking, you’ll overlook indications of difficulties till it’s much too late. But with the best resources set up, you remain on top of things.
In brief, checking assists you keep the app responsible and scalable. It’s not nearly recognizing failures—it’s about comprehension your system and making certain it works properly, even stressed.
Last Views
Scalability isn’t just for major businesses. Even compact apps have to have a powerful Basis. By designing meticulously, optimizing sensibly, and using the appropriate tools, it is possible to Establish apps that increase effortlessly without having breaking stressed. Start tiny, Assume big, and Construct clever.